GSoC Week 7: Completing the Gameplay Loop
Posted on July 4th, 2018
So far I've been working on getting the bare bones working for the main Capture the Flag gameplay. This week I worked on more improvements to this, to get the game into a more playable state.
Firstly, I focused to improvements for having players drop and pick up the flag. Before, when a player tagged another, the other player would only drop the flag they had if it was being held. I instead search through the player's inventory to see if there is a flag in there, and if so, it is dropped.
I did this by creating a loop that searched the player's inventory for the appropriate item:
// AttackSystem.java
int inventorySize = inventoryManager.getNumSlots(entity);
for (int slotNumber = 0; slotNumber <= inventorySize; slotNumber++) {
EntityRef inventorySlot = inventoryManager.getItemInSlot(entity, slotNumber);
if (inventorySlot.hasComponent(BlockItemComponent.class)) {
if (inventorySlot.getComponent(BlockItemComponent.class).blockFamily.getURI().toString().equals(flagTeam)) {
return inventorySlot;
}
}
}
return EntityRef.NULL;
And then taking the appropriate action, for instance if an inventorySlot with a red flag is found:
// AttackSystem.java
if (redFlagSlot != EntityRef.NULL) {
Vector3f position = new Vector3f(entity.getComponent(LocationComponent.class).getLocalPosition());
Vector3f impulseVector = new Vector3f(attackingPlayer.getComponent(LocationComponent.class).getLocalPosition());
entity.send(new DropItemRequest(redFlagSlot, entity, impulseVector, position));
return;
}
I also added code to make the flag teleport back to the base on score increase:
// ScoreSystem.java
// On Black team scoring, return captured red flag to base
inventoryManager.removeItem(player, player, heldItem, true);
worldProvider.setBlock(new Vector3i(LASUtils.CENTER_RED_BASE_POSITION.x, LASUtils.CENTER_RED_BASE_POSITION.y + 1, LASUtils.CENTER_RED_BASE_POSITION.z), blockManager.getBlock(LASUtils.RED_FLAG_URI));
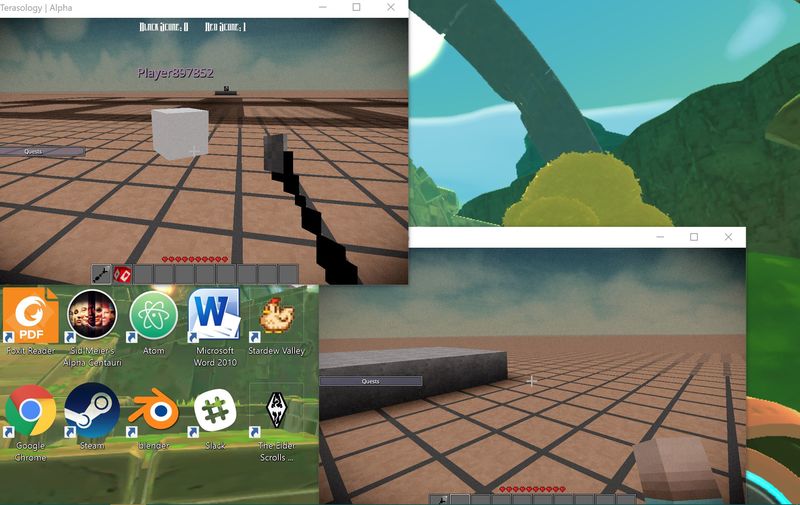
Two players face off
Finally I worked on reskinning the UI to make it look better and fit into the game mode for Light And Shadow. I used the same font used for some of the Light And Shadow material, and used dark gray and red colors for parts of the score UI.
I created a new .skin file for the UI, and reskinned it like so:
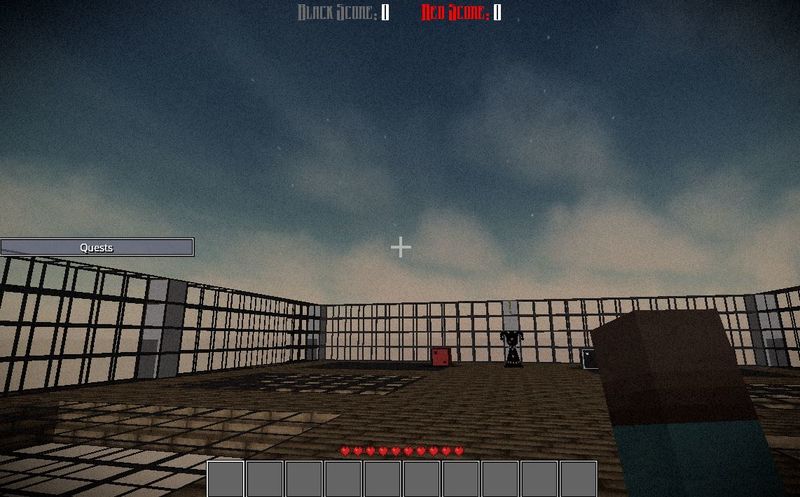
Also want to give a special thanks for the folks at Terasology for taking the module out for a test drive, doing some great multiplayer gaming and taking notes of things that needed changes.
So far so good! With the changes from this week, the Capture the Flag game mode is now far more playable and fun.
There are still some bugs that need to be worked out, mainly issues with things not being consistent across multiplayer like the score UI and Magic Fool NPC.
I also want to get started on some of the final things that will make the game mode complete, the big things being adding a particle effect to players carrying the flag, and handling the end of the game, after multiple rounds are won--resetting the world.
So far, I feel I've been making good headway, and things are really coming along now. Looking forward to seeing this come together even more.
PRs for this week:
LAS: https://github.com/Terasology/LightAndShadow/pull/69
LASR: https://github.com/Terasology/LightAndShadowResources/pull/28