GSoC Week 11: Nearing the End
Posted on August 2nd, 2018
It's hard to believe that Google Summer of Code is almost over!
This week I focused mainly on getting the particle effects for when the player is holding the flag working across multiplayer, so that the host and any joined clients were in sync. One of the problems with the code is that the adding and removing of the particle effects happened in a lot of different places--when the player was attacked, when the player pressed `E` to pick up a flag, etc. I got rid of this redundancy by just having the server send out an event to all clients when the flag was added or removed from the player's inventory. Because the call was made multiple times, I had to check whether or not the player already had a FlagParticleComponent on them before adding it, otherwise an incorrect number of particle entities would spawn in the world.
// In ClientParticleSystem, an example of handling flag pickup through events
// Much of the creating a child particle component comes from here: https://gist.github.com/jellysnake/2a18b1670fe26b59cbdae7c686356cde
@ReceiveEvent
public void onFlagPickup(FlagPickupEvent event, EntityRef entity) {
String team = event.team;
EntityRef player = event.player;
if (!player.hasComponent(FlagParticleComponent.class)) {
EntityRef particleEntity = entityManager.create(LASUtils.getFlagParticle(team));
FlagParticleComponent particleComponent = new FlagParticleComponent(particleEntity);
LocationComponent targetLoc = player.getComponent(LocationComponent.class);
LocationComponent childLoc = particleEntity.getComponent(LocationComponent.class);
childLoc.setWorldPosition(targetLoc.getWorldPosition());
Location.attachChild(player, particleEntity);
particleEntity.setOwner(player);
player.addOrSaveComponent(particleComponent);
}
if (!player.hasComponent(HasFlagComponent.class)) {
player.addComponent(new HasFlagComponent(team));
}
}
I also worked on some smaller improvements to gameplay, such as changing the texture on the bases to red and black respectively, and changing the flag drop on player attack to land closer to the midpoint between the attacker and the target, so it's easier for either party to take the flag.
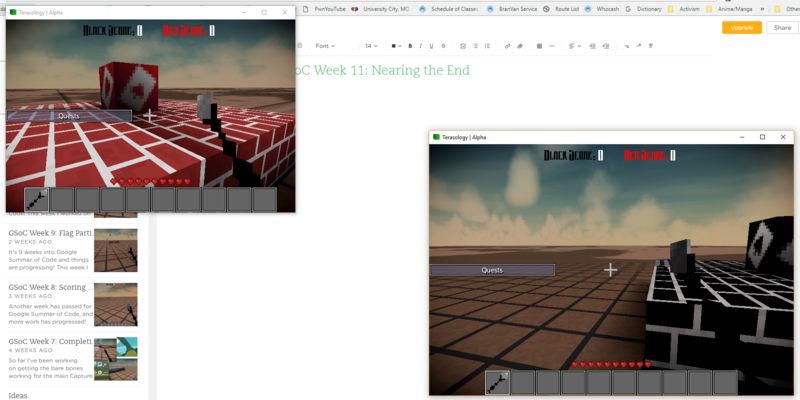
Different bases for different teams
There's still a number of things I want to get done before the big playtest and code wrap up:
+ Properly handling player skinning, with player skins appearing correctly rotated and player cube removed
+ Handling one team winning (reaching max score) -- there's a lot that needs to go into a level reset, but I at least want to add something to indicate this, such as some UI text popping up to show which team won
+ UI indication of which team a player is on when they choose a team
+ Ideally, still putting in a maze to make the CTF gameplay more challenging
So I'll be trying to get everything good and ready for the end of GSoC!
PRs for this week:
Adding Client HUD and Score System: https://github.com/Terasology/LightAndShadow/pull/70
Changes base blocks to be red/black depending on base instead of gray stone: https://github.com/Terasology/LightAndShadowResources/issues/30